Why TypeScript
One language to rule them all (and to anything)
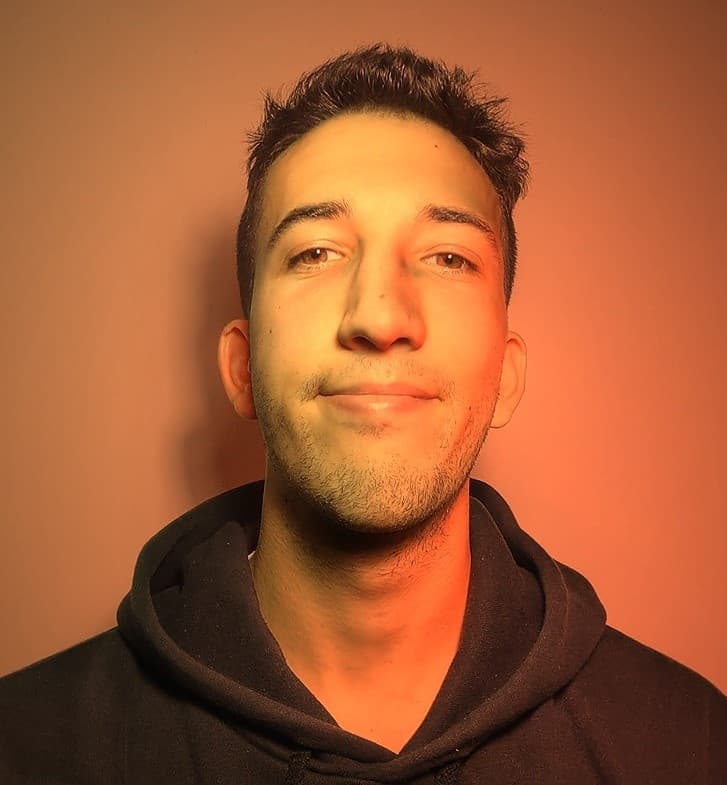
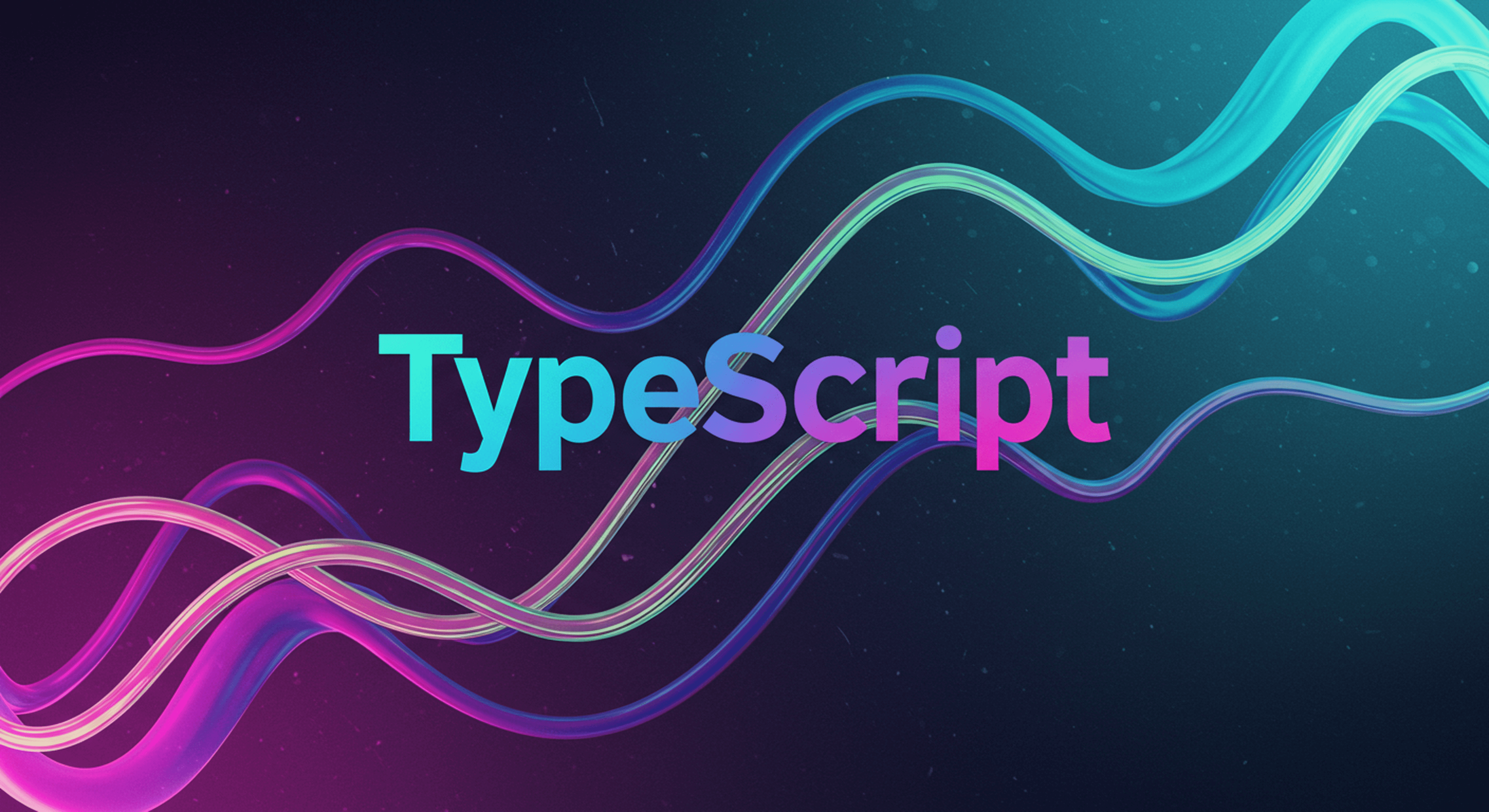
Why TypeScript: My Full-stack Codebase of Choice
TypeScript has become my go-to language for building full-stack applications. After years of working with various languages and frameworks, I've settled on a TypeScript-powered stack that delivers exceptional developer experience, maintainability, and performance.
In this post, I'll explain why TypeScript forms the backbone of my development workflow and how my preferred stack — Next.js, Drizzle ORM, and PostgreSQL — creates a powerful foundation for modern SaaS applications. 🚀
🤔 Why TypeScript Over Plain JavaScript?
JavaScript's flexibility is both its strength and weakness. While it allows for rapid development, this same flexibility can lead to subtle bugs and maintenance challenges as projects grow. TypeScript addresses these issues by adding:
✅ Type Safety
Catches type-related errors at compile time instead of runtime
// JavaScript
function getUser(id) {
// What type is id?
// What does this return?
return fetchUser(id);
}
// TypeScript
function getUser(id: string): Promise<User> {
return fetchUser(id);
}
📚 Better Documentation
Types serve as built-in documentation that stays updated
interface UserSettings {
notifications: {
email: boolean;
push: boolean;
sms?: boolean;
};
theme: 'light' | 'dark' | 'system';
timezone: string;
}
⚡ Enhanced IDE Support
Rich autocompletion, inline errors, and refactoring tools
🔄 Safer Refactoring
Rename properties and functions with confidence
⚙️ My Full-Stack TypeScript Architecture
For SaaS applications, my stack of choice centers around TypeScript with these key technologies:
🔄 Next.js: Unified Frontend and Backend
Next.js allows me to build both the frontend and backend with TypeScript in a single codebase. This unified approach offers:
- Type consistency across client and server — shared interfaces and validation logic
- Simplified deployment — one repository to manage
- Multiple rendering strategies — SSR, SSG, and client-side rendering as needed
- Optimized performance — automatic code splitting and server components
💧 Drizzle ORM: TypeScript-First Database Access
For database access, I've moved from Prisma to Drizzle ORM, a lightweight TypeScript-first ORM that offers:
- Type-safe queries — compiler catches SQL errors
- Superior performance — no runtime overhead compared to other ORMs
- SQL-like syntax — familiar for those with SQL experience
- Schema migrations — version control for your database schema
Here's how Drizzle keeps your database operations type-safe:
// Define your schema with TypeScript
import { pgTable, serial, text, boolean, timestamp } from 'drizzle-orm/pg-core';
export const users = pgTable('users', {
id: serial('id').primaryKey(),
name: text('name').notNull(),
email: text('email').notNull().unique(),
isActive: boolean('is_active').default(true),
createdAt: timestamp('created_at').defaultNow()
});
// Type-safe queries
const newUser = await db.insert(users)
.values({
name: 'Michele',
email: 'michele.vigano.work@gmail.com',
// TypeScript error: Missing required fields or wrong types
})
.returning();
// Type-safe selections
const activeUsers = await db.select({
id: users.id,
name: users.name
})
.from(users)
.where(eq(users.isActive, true));
// activeUsers is typed as { id: number; name: string }[]
🐘 PostgreSQL: The Reliable Foundation
For most projects, I choose PostgreSQL as my database because it offers:
- Robust reliability — mature, battle-tested database engine
- Rich feature set — from JSON support to full-text search
- Excellent TypeScript support via Drizzle ORM
- Scalability options — from single instance to clustered deployments
🔍 Type Safety Across the Full Stack
The true power of this architecture is end-to-end type safety. Types defined in one layer can be used throughout the application:
// shared/types.ts - Define once, use everywhere
import { InferSelectModel } from 'drizzle-orm';
import { users } from '../db/schema';
export type User = InferSelectModel<typeof users>;
// API route
import { User } from '@/shared/types';
export async function GET(): Promise<Response> {
const users: User[] = await db.select().from(users);
return Response.json(users);
}
// React component
import { User } from '@/shared/types';
function UserList({ users }: { users: User[] }) {
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
🚀 Why Node.js Over Python?
For web applications, I prefer Node.js with TypeScript over Python for several reasons:
Consideration | Node.js + TypeScript | Python |
---|---|---|
Language Consistency | ✅ Same language front-to-back | ❌ Different languages front/back |
Performance | ✅ Generally faster for web workloads | ⚠️ Typically slower for IO-bound operations |
Type Safety | ✅ Strong with TypeScript | ⚠️ Limited with type hints |
Async Model | ✅ Built for asynchronous operations | ⚠️ Added via asyncio |
🧪 Real-world Results
After building multiple SaaS applications with this stack, I've observed these benefits:
- Fewer production bugs — Static typing catches issues before deployment
- Faster development iterations — IDE support accelerates coding
- Improved collaboration — Team members can understand code faster
- Better refactoring confidence — Change code without breaking functionality
- More maintainable codebases — Clarity and consistency across the stack
🎯 Conclusion: The TypeScript Advantage
While no technology stack is perfect for every scenario, TypeScript with Next.js, Drizzle ORM, and PostgreSQL has proven to be a robust, maintainable, and efficient combination for the SaaS applications I build. The end-to-end type safety and developer experience benefits have consistently translated into higher quality products delivered in less time.
If you're considering your next project's architecture, I highly recommend evaluating this TypeScript-powered stack. The initial learning curve is well worth the long-term benefits in code quality, maintainability, and developer productivity.